Data types are one of the more tedious and uninteresting parts about programming, at least your goal is to complete a project rather than understand the intricacies of… data types. But a basic understanding is needed.
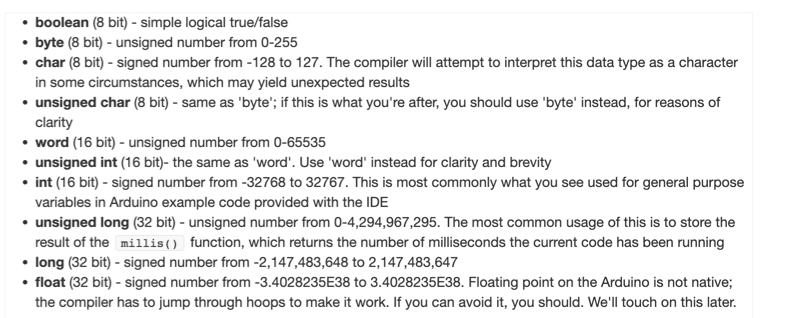
The important takeaway is the last line: “Floating point math on the Arduino is not native and should be avoided”
Floating point / Floats are…… numbers with decimals, like 1.1111.
I try to always use Int’s (integers between -32,768 and +32,768) (Int’s can’t have decimal places) when doing math, and only convert to Floats for display purposes. This isn’t so bad, because most all sensor data comes into the program as Int’s.
Sometimes this is inconvenient, I’m too lazy, and I ignore this advice. It has never caused any problems, but surely there’s increased risk if running more challenging programs or using more memory (I’m not sure which).
Strings
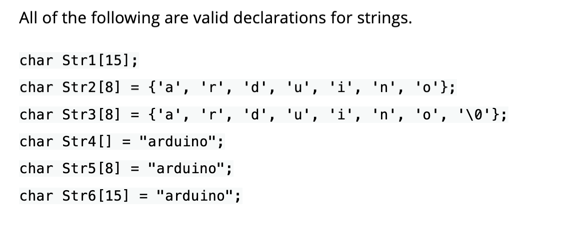
Notice that arduino has 7 letters yet 8 spaces are needed, to account for the terminator ‘\0’
Strings are always defined inside double quotes (“Abc”) and characters are always defined inside single quotes(‘A’).
Pointers
A pointer is a variable that stores the address of another variable. This is helpful, for example, for passing around large chunks of data without having to copy them When creating a pointer, use the * operator before the name.
The * operator can also be used to get the contents of the address that a pointer is pointing at. (Think of *px as “the thing stored at address px)
Use the “&” symbol to say “the address of”.
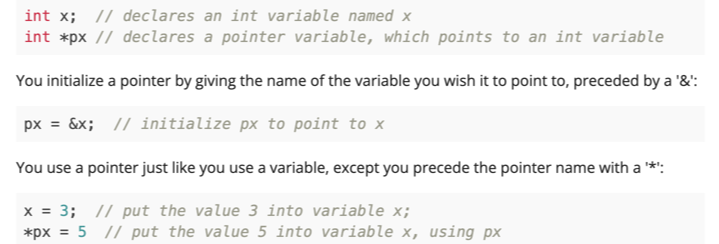
In C and C++ arrays are basically pointers too.
An array is a collection of variables that are accessed with an index number. The elements of an array are stored at contiguous memory locations.
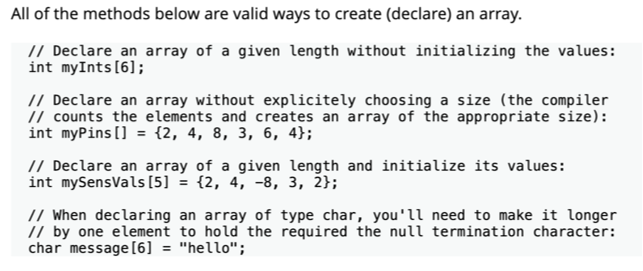
Wherever you have an array you can use it as a pointer and vice versa. You’ll
see this all over the place for char * strings, perhaps the commonest use of pointers.
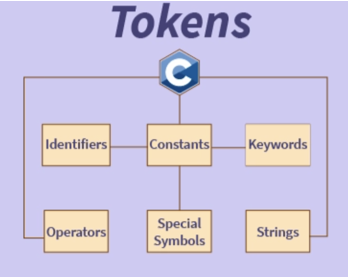
Tokens are relevant because the return of the strtok function, commonly used in reading serial data, is a Pointer to a Token. Accessing the actual token data is not trivial, took me a whole day to figure out, will be the subject of a future page.